Basic Syntax
Template Interpolation
<div id="app">
<p>๋ฉ์์ง: {{ msg }}</p>
<p>HTML ๋ฉ์์ง : {{ rawHTML }}</p>
<p>HTML ๋ฉ์์ง : <span v-html="rawHTML"></span></p>
<p>{{ msg.split('').reverse().join('') }}</p>
</div>
<script>
// 1. Text interpolation
const app = new Vue({
el: "#app",
data: {
msg: "Text interpolation",
rawHTML: '<span style="color:red"> ๋นจ๊ฐ ๊ธ์จ</span>',
},
});
</script>
โ ๊ฐ์ฅ ๊ธฐ๋ณธ์ ์ธ ๋ฐ์ธ๋ฉ(์ฐ๊ฒฐ) ๋ฐฉ๋ฒ
โ ์ค๊ดํธ 2๊ฐ๋ก ํ๊ธฐ
Directives
๊ธฐ๋ณธ ๊ตฌ์ฑ
โ v-์ ๋์ฌ๊ฐ ์๋ ํน์ ์์ฑ์๋ ๊ฐ์ ํ ๋นํ ์ ์๋ค.
- ๊ฐ์๋ JSํํ์์ ์์ฑํ ์ ์์
โ directive์ ์ญํ ์ ํํ์์ ๊ฐ์ด ๋ณ๊ฒฐ๋ ๋ ๋ฐ์์ ์ผ๋ก DOM์ ์ฐ๊ฒฐํ๋ ๊ฒ
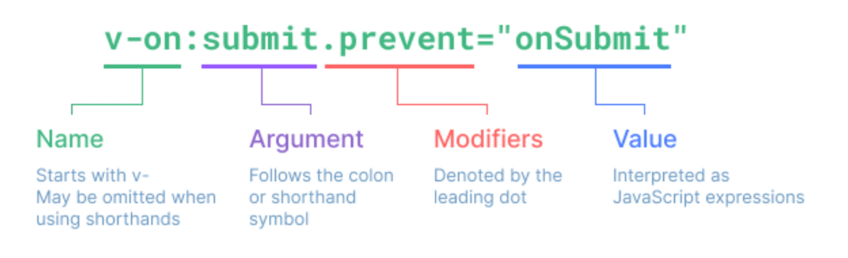
โ :
์ ํตํด ์ ๋ฌ์ธ์๋ฅผ ๋ฐ์ ์ ์์
โ .
์ผ๋ก ํ์๋๋ ํน์ ์ ๋ฏธ์ฌ directive์ ํน๋ณํ ๋ฐฉ๋ฒ์ผ๋ก ๋ฐ์ธ๋ฉ
v-text & v-html
<div id="app2">
<!-- v-text & {{}} -->
<p v-text="message"></p>
<!-- ๋์ผ -->
<p>{{ message }}</p>
</div>
const app2 = new Vue({
el: "#app2",
data: {
// text
message: "Hello!",
// html
html: '<a href="https://www.google.com">GOOGLE</a>',
},
});
โ Template interpolation๊ณผ ํจ๊ป ๊ฐ์ฅ ๊ธฐ๋ณธ์ ์ธ ๋ฐ์ธ๋ฉ ๋ฐฉ๋ฒ
โ v-html
- RAW HTML์ ํํํ ์ ์๋ ๋ฐฉ๋ฒ
- ๋จ, ์ฌ์ฉ์๊ฐ ์ ๋ ฅํ๊ฑฐ๋ ์ ๊ณตํ๋ ์ปจํ ์ธ ์๋ ์ ๋ ์ฌ์ฉ ๊ธ์ง (XSS ๊ณต๊ฒฉ)
v-show & v-if
<div id="app3">
<p v-show="isActive">๋ณด์ด๋? ์๋ณด์ด๋?</p>
<p v-if="isActive">์๋ณด์ด๋? ๋ณด์ด๋?</p>
</div>
const app3 = new Vue({
el: "#app3",
data: {
isActive: false,
},
});
โ v-show
- ํํ์์ ์์ฑ๋ ๊ฐ์ ๋ฐ๋ผ element๋ฅผ ๋ณด์ฌ์ค ๊ฒ์ธ์ง ๊ฒฐ์ (boolean ๊ฐ์ด ๋ณ๊ฒฝ๋ ๋๋ง๋ค ๋ฐ์)
- ๋์ element์ display์์ฑ์ ๊ธฐ๋ณธ ์์ฑ๊ณผ none์ผ๋ก toggle
- ์์ ์์ฒด๋ ํญ์ DOM์ ๋ ๋๋ง ๋๋ค.
โ v-if
- v-show์ ์ฌ์ฉ ๋ฐฉ๋ฒ์ ๋์ผ
- ๊ฐ์ด false์ธ ๊ฒฝ์ฐ DOM์์ ์ฌ๋ผ์ง
v-show vs v-if
โ v-show(Expensive initial load, cheap toggle)
- ํํ์ ๊ฒฐ๊ณผ์ ๊ด๊ณ์์ด ๋ ๋๋ง ๋๋ฏ๋ก ์ด๊ธฐ ๋ ๋๋ง์ ํ์ํ ๋น์ฉ์ v-if๋ณด๋ค ๋์ ์ ์์
- display ์์ฑ ๋ณ๊ฒฝ์ผ๋ก ํํ ์ฌ๋ถ๋ฅผ ํ๋จํ๋ฏ๋ก ๋ ๋๋ง ํ toggle ๋น์ฉ์ ์ ์
โ v-if (Cheap initial load, expensive toggle)
- ํํ์ ๊ฒฐ๊ณผ๊ฐ false์ธ ๊ฒฝ์ฐ ๋ ๋๋ง์กฐ์ฐจ ๋์ง ์์ผ๋ฏ๋ก ์ด๊ธฐ ๋ ๋๋ง ๋น์ฉ์ ๋ฎ์ ์ ์์
- ๋จ, ํํ์ ๊ฐ์ด ์์ฃผ ๋ณ๊ฒฝ๋๋ ๊ฒฝ์ฐ ์ฆ์ ์ฌ ๋ ๋๋ง์ผ๋ก ๋น์ฉ์ด ์ฆ๊ฐ
- else, if-else ๋ฑ๊ณผ ํจ๊ป ์กฐ๊ฑด๋ฌธ์ผ๋ก ์ด์ฉ
v-for
- string
<div id="app">
<h2>String</h2>
<div v-for="char in myStr">{{ char }}</div>
<div v-for="(char, index) in myStr" :key="index">
<p>{{ index }}๋ฒ์งธ ๋ฌธ์์ด {{ char }}</p>
</div>
</div>
<script>
const app = new Vue({
el: "#app",
data: {
// 1. String
myStr: "Hello, World!",
},
});
</script>
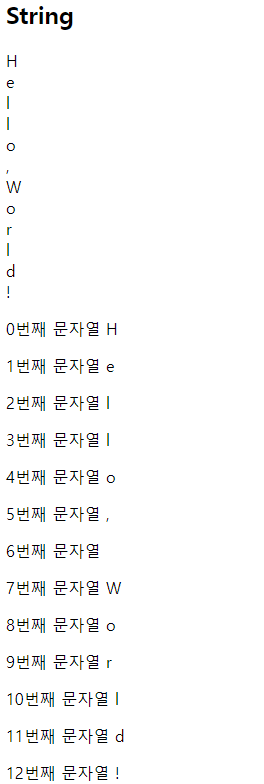
- array
<h2>Array</h2>
<div v-for="(item, index) in myArr" :key="index">
<p>{{ index }}๋ฒ์งธ ์์ดํ
{{ item }}</p>
</div>
<div v-for="(item, index) in myArr2" :key="`arry-${index}`">
<p>{{ index }}๋ฒ์งธ ์์ดํ
</p>
<p>{{ item.name }}</p>
</div>
<script>
const app = new Vue({
el: "#app",
data: {
// 2-1. Array
myArr: ["python", "django", "vue.js"],
// 2-2. Array with Object
myArr2: [
{ id: 1, name: "python", completed: true },
{ id: 2, name: "django", completed: true },
{ id: 3, name: "vue.js", completed: false },
],
},
});
</script>

- objects
<h2>Object</h2>
<div v-for="value in myObj">
<p>{{ value }}</p>
</div>
<div v-for="(value, key) in myObj" :key="key">
<p>{{ key }} : {{ value }}</p>
</div>
<script>
const app = new Vue({
el: "#app",
data: {
// 3. Object
myObj: {
name: "sun",
age: 27,
},
},
});
</script>

โ for.. in..
ํ์์ผ๋ก ์์ฑ
โ ๋ฐ๋ณตํ๋ ๋ฐ์ดํฐ ํ์
์ ๋ชจ๋ ์ฌ์ฉ ๊ฐ๋ฅ
โ index๋ฅผ ํจ๊ป ์ถ๋ ฅํ๊ณ ์ ํ๋ค๋ฉด (char, index)
ํํ๋ก ์ฌ์ฉ ๊ฐ๋ฅ
ํน์ ์์ฑ key
โ v-for ์ฌ์ฉ ์ ๋ฐ๋์ key ์์ฑ์ ๊ฐ ์์์ ์์ฑ
โ ์ฃผ๋ก v-for directive ์์ฑ ์ ์ฌ์ฉ
โ vue ํ๋ฉด ๊ตฌ์ฑ ์ ์ด์ ๊ณผ ๋ฌ๋ผ์ง ์ ์ ํ์ธํ๋ ์ฉ๋๋ก ํ์ฉ
- ๋ฐ๋ผ์ key ์ค๋ณต x
โ ๊ฐ ์์๊ฐ ๊ณ ์ ํ ๊ฐ์ ๊ฐ์ง๊ณ ์๋ค๋ฉด ์๋ต ๊ฐ๋ฅ
v-on
โ :
์ ํตํด ์ ๋ฌ๋ฐ์ ์ธ์๋ฅผ ํ์ธ
โ ๊ฐ์ผ๋ก JSํํ์ ์์ฑ
โ addEventListener
์ ์ฒซ๋ฒ์งธ ์ธ์์ ๋์ผํ ๊ฐ๋ค๋ก ๊ตฌ์ฑ
โ ๋๊ธฐํ๊ณ ์๋ ์ด๋ฒคํธ๊ฐ ๋ฐ์ํ๋ฉด ํ ๋น๋ ํํ์ ์คํ
<body>
<div id="app">
<button v-on:click="number++">increase Number</button>
<p>{{ number }}</p>
</div>
...
</body>
<script>
const app = new Vue({
el: "#app",
data: {
number: 0,
isActive: false,
},
});
</script>
โ method๋ฅผ ํตํ data ์กฐ์๋ ๊ฐ๋ฅ
โ method์ ์ธ์๋ฅผ ๋๊ธฐ๋ ๋ฐฉ๋ฒ์ ์ผ๋ฐ ํจ์๋ฅผ ํธ์ถํ ๋์ ๋์ผํ ๋ฐฉ์
โ :
์ ํตํด ์ ๋ฌ๋ ์ธ์์ ๋ฐ๋ผ ํน๋ณํ modifiers(์์์ด)๊ฐ ์์ ์ ์์
- ex)
v-on:keyup.enter
โ @
shortcut ์ ๊ณต
- ex)
@keyup.click
<body>
<div id="app">
...
<button v-on:click="toggleActive">toggle isActive</button>
<button @click="checkActive(isActive)">check isActive</button>
<p>{{ isActive }}</p>
</div>
</body>
<script>
const app = new Vue({
el: "#app",
data: {
number: 0,
isActive: false,
},
methods: {
toggleActive: function () {
this.isActive = !this.isActive;
},
checkActive: function (check) {
console.log(check);
},
},
});
</script>
v-bind
<div id="app2">
<a v-bind:href="url">Go To GOOGLE</a>
<p v-bind:class="redTextClass">๋นจ๊ฐ ๊ธ์จ</p>
<p v-bind:class="{ 'red-text': true }">๋นจ๊ฐ ๊ธ์จ</p>
<p v-bind:class="[redTextClass, borderBlack]">๋นจ๊ฐ ๊ธ์จ, ๊ฒ์ ํ
๋๋ฆฌ</p>
<!-- : shortcut -->
<p :class="theme">์ํฉ์ ๋ฐ๋ฅธ ํ์ฑํ</p>
<button @click="darkModeToggle">dark Mode {{ isActive }}</button>
</div>
<script>
const app2 = new Vue({
el: "#app2",
data: {
url: "https://www.google.com/",
redTextClass: "red-text",
borderBlack: "border-black",
isActive: true,
theme: "dark-mode",
},
methods: {
darkModeToggle() {
this.isActive = !this.isActive;
if (this.isActive) {
this.theme = "dark-mode";
} else {
this.theme = "white-mode";
}
},
},
});
</script>
โ HTML ๊ธฐ๋ณธ ์์ฑ์ Vue data๋ฅผ ์ฐ๊ฒฐ
โ class์ ๊ฒฝ์ฐ ๋ค์ํ ํํ๋ก ์ฐ๊ฒฐ ๊ฐ๋ฅ
โ ์กฐ๊ฑด๋ถ ๋ฐ์ธ๋ฉ
- `{'class Name': '์กฐ๊ฑด ํํ์'}
- ์ผํญ ์ฐ์ฐ์ ๊ฐ๋ฅ
โ ๋ค์ค ๋ฐ์ธ๋ฉ
['JSํํ์', 'JSํํ์',...]
โ :
shortcut ์ ๊ณต
v-model
<div id="app">
<h2>1. Input -> Data</h2>
<h3>{{ myMessage }}</h3>
<input @input="onInputChange" type="text" />
<hr />
<h2>2. Input <-> Data</h2>
<h3>{{ myMessage2 }}</h3>
<input v-model="myMessage2" type="text" />
<hr />
</div>
<script>
const app = new Vue({
el: "#app",
data: {
myMessage: "",
myMessage2: "",
},
methods: {
onInputChange: function (event) {
this.myMessage = event.target.value;
},
},
});
</script>
โ Vue instance์ DOM์ ์๋ฐฉํฅ ๋ฐ์ธ๋ฉ
โ Vue data ๋ณ๊ฒฝ ์ v-model๋ก ์ฐ๊ฒฐ๋ ์ฌ์ฉ์ ์
๋ ฅ element์๋ ์ ์ฉ
โ ํ๊ธ, ์ผ๋ณธ์ด, ์ค๊ตญ์ด ์์ ํ ์ง์ x
'โญ Personal_Study > Vue' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
SFC (Single File Component) (0) | 2022.11.11 |
---|---|
Vue_CLI (0) | 2022.11.09 |
Vue: advanced attributes (0) | 2022.11.07 |
Vue Instance (0) | 2022.11.06 |
Vue Intro (0) | 2022.11.06 |
๋๊ธ